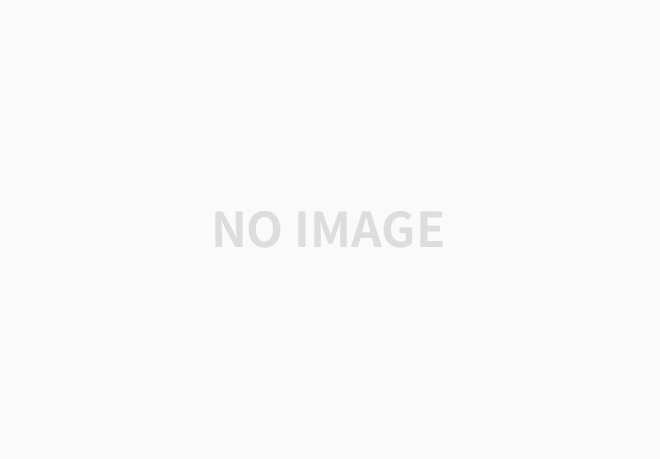
๐ผ ํด๋์ค์ ๊ธฐ๋ณธ ๋ฌธ๋ฒ
class ClassName {
constructor() { }
method1() { }
method2() { }
method3() { }
}
ํด๋์ค๋ฅผ ์์ฑํ ๋ค 'new ClassName()'์ ํธ์ถํ๋ฉด ๊ฐ์ฒด๊ฐ ์์ฑ๋๋ค.
constructor๋ new์ ์ํด ์๋์ผ๋ก ํธ์ถ๋๋ฉฐ, ๊ฐ์ฒด๋ฅผ ์ด๊ธฐํํ ์ ์๋ค.
class Fruit {
constructor(name) {
this.name = name;
}
say() {
console.log(this.name);
}
}
const apple = new Fruit('apple');
apple.say(); //apple
new Fruit๋ฅผ ํ๋ ์๊ฐ ์๋ก์ด ๊ฐ์ฒด๊ฐ ์์ฑ๋๊ณ constructor๊ฐ ์๋์ผ๋ก ์คํ๋๋ค.
this.name์ 'apple'์ด ํ ๋น๋๊ณ , ๋ฉ์๋ say๋ฅผ ์คํํ๋ฉด 'apple'์ด ์ถ๋ ฅ๋๋ค.
๐ผ getter์ setter
class์์๋ ์ ๊ทผ์ ํ๋กํผํฐ์ธ get๊ณผ set์ ์ฌ์ฉํด ๋ค๋ฅธ ๋ฐ์ดํฐ์ ํ๋กํผํฐ ๊ฐ์ ์ฝ๊ฑฐ๋ ์ ์ฅํ ์ ์๋ค.
๋ ๋ค ๋ฉ์๋ ์ด๋ฆ ์์ get, set ํค์๋๋ฅผ ๋ถ์ฌ ์ฌ์ฉํ๋ค.
class Student {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.lastName}${this.firstName}`;
}
set fullName(value) {
console.log(value);
}
}
const student = new Student('๊ธธ๋', 'ํ');
console.log(student.fullName);
get์ ๊ฐ์ ธ์ค๋ ์ฉ๋์ด๋ฏ๋ก return์ด ํ์ํ๊ณ
set์ ํ๋กํผํฐ์ ํ ๋นํ๋ ์ฉ๋์ด๋ฏ๋ก ๋งค๊ฐ๋ณ์๊ฐ ์์ด์ผ ํ๋ค.
๐ผ ํด๋์ค ํ๋
ํด๋์ค ํ๋๋ฅผ ์ฌ์ฉํ๋ฉด ์ด๋ค ์ข ๋ฅ์ ํ๋กํผํฐ๋ ํด๋์ค์ ์ถ๊ฐํ ์ ์๋ค.
class Student {
name = "๊ธธ๋";
greeting() {
console.log(`${this.name} ์๋
!`);
}
}
new Student().greeting(); // ๊ธธ๋ ์๋
!
ํด๋์ค ํ๋๋ 'ํ๋กํผํฐ ์ด๋ฆ = ๊ฐ'์ ์ฐ๋ฉด ๋ง๋ค ์ ์๋ค.
ํด๋์ค ํ๋๋ ๊ฐ๋ณ ๊ฐ์ฒด์๋ง ํด๋์ค ํ๋๊ฐ ์ค์ ๋๋ค.
๋ฐ๋ผ์ Student.prototype์๋ ์ค์ ๋์ง ์๋๋ค.
class Student {
name = "๊ธธ๋";
}
const student = new Student();
console.log(student.name); //๊ธธ๋
console.log(Student.prototype.name); //undefined
๐ผ ์ ๊ทผ ์ ์ด์
์๋ฐ์คํฌ๋ฆฝํธ๋ ๊ธฐ๋ณธ์ ์ผ๋ก public์ด๋ค.
pubilc ํ๋๋ ์ด๋์๋ ์ฐธ์กฐํ ์ ์๋ค.
์ด๋ฐ ๊ฒฝ์ฐ ๋๊ตฌ๋ ์์ฝ๊ฒ ์ํ๋ ๊ฐ์ ์ ๊ทผํด ๋ณ๊ฒฝ์ ๊ฐํ ์ ์๋ ์ํ์ด ์๋ค.
๋ฐ๋ผ์ private์ผ๋ก ๋ง๋ค์ด ๋ฐ์์ ์ ๊ทผํ ์ ์๊ฒ ๋ง๋ ๋ค.
'#'์ ๋ถ์ฌ ์ฌ์ฉํ๊ณ ํด๋์ค ์์์๋ง ์ ๊ทผ์ด ๊ฐ๋ฅํ๋ค.
class Fruit {
#name;
constructor(name) {
this.#name = name;
}
}
const apple = new Fruit('apple');
apple.#name = 'orange';
console.log(apple); //SyntaxError: Private field '#name' must be declared in an enclosing class
ํ๋กํผํฐ๊ฐ ์ ์๋ ํด๋์ค์ ๊ทธ ํด๋์ค๋ฅผ ์์๋ฐ๋ ํด๋์ค์์๋ง ์ฌ์ฉ๊ฐ๋ฅํ protected๋ ์๋ค.
protected ์ญ์ private์ฒ๋ผ ๋ด๋ถ ์ธํฐํ์ด์ค๋ฅผ ์จ๊ธธ ๋ ์ฌ์ฉํ๋ค.
'_'๋ก ์์ํ๋๋ฐ ์๋ฐ์คํฌ๋ฆฝํธ์์ ์ง์ํ๋ ๋ฌธ๋ฒ์ ์๋๊ณ ๊ด์ต์ ์ผ๋ก ์ฌ์ฉํ๋ค.
๐ผ ์ ์ ๋ฉ์๋์ ์ ์ ํ๋กํผํฐ
prototype์ด ์๋ ํด๋์ค ํจ์ ๋ด์์ ๋ฉ์๋์ ํ๋กํผํฐ๋ฅผ ๋ง๋ค ์ ์๋ค.
์์ฑ์ ํจ์๋ก ์ธ์คํด์ค๋ฅผ ์์ฑํ์ง ์์๋ ์ฐธ์กฐ/ํธ์ถํ ์ ์๋ค.
class Student {
static staticMethod() {
console.log('static');
}
}
Student.staticMethod(); // static
const student = new Student();
student.staticMethod(); //TypeError
์ ์ ํ๋กํผํฐ์ ์ ์ ๋ฉ์๋๋ ์์ฑ์ ํจ์๊ฐ ์์ฑํ ์ธ์คํด์ค๋ก ์ฐธ์กฐ/ํธ์ถํ ์ ์๋ค.
๋ํ, ํด๋์ค ๋ ๋ฒจ์ ๋ฉ์๋์์๋ this๋ฅผ ์ฐธ์กฐํ ์ ์๋ค.
class Product {
constructor(name, expirationDate) {
this.name = name;
this.expirationDate = expirationDate;
}
static compareExpirationDate(product1, product2) {
return product1.expirationDate - product2.expirationDate;
}
}
let products = [
new Product('apple', 2),
new Product('banana', 3),
new Product('orange', 1),
new Product('lemon', 5),
];
products.sort(Product.compareExpirationDate);
console.log(products);
// [
// Product { name: 'orange', expirationDate: 1 },
// Product { name: 'apple', expirationDate: 2 },
// Product { name: 'banana', expirationDate: 3 },
// Product { name: 'lemon', expirationDate: 5 }
// ]
Product.compareExpirationDate๋ products๋ฅผ ๋น๊ตํด ์ฃผ๋ ์ญํ ์ ํ๋ค.
๋ฐ๋ผ์ ์์์ ๋ฐ๋ผ๋ณด๋ ๋ฐฉ์์ผ๋ก ์ญํ ์ ์ํํด์ผ ํ๋ค.
๊ทธ๋์ ๊ฐ products๋ค์ expiration date๋ฅผ ๋น๊ตํ ์ ์๋ ๊ฒ์ด๋ค.
๐ผ ์์
ํด๋์ค๋ ์์ํ ์ ์๋ค.
์์์ ์ฌ์ฉํ๋ฉด ๋ค๋ฅธ ํด๋์ค๋ก์ ํ์ฅ์ด ๊ฐ๋ฅํด์ง๋ค.
class Dog {
constructor(name) {
this.name = name;
}
eat() {
console.log('๋จน๋๋ค');
}
play() {
console.log('๋
ผ๋ค');
}
}
class Person {
constructor(name) {
this.name = name;
}
eat() {
console.log('๋จน๋๋ค');
}
play() {
console.log('๋
ผ๋ค');
}
drink(){
console.log('์ ๋ง์ ๋ค');
}
}
๋๊ฐ์ ํด๋์ค๊ฐ ์๋ค.
ํ๋๋ ๊ฐ์์ง, ํ๋๋ ์ฌ๋์ด๋ค.
๋ ๋ค ์ด๋ฆ์ด ์๊ณ , ๋จน๊ณ , ๋ ผ๋ค.
๋ค๋ง ๋ค๋ฅธ ๊ฒ์ ์ฌ๋์ ์ ์ ๋ง์ ๋ค๋ ๊ฒ์ด๋ค.
class Dog์ Person์ ์ค๋ณต๋๋ ๊ฒ์ด ๋๋ฌด ๋ง๋ค.
์ด๋, ์์์ ์ฌ์ฉํ๋ฉด ๋๋ค.
class Animal {
constructor(name) {
this.name = name;
}
eat() {
console.log('๋จน๋๋ค');
}
play() {
console.log('๋
ผ๋ค');
}
}
class Dog extends Animal {}
const dog = new Dog('๋ฐ๋์ด');
dog.play();
class Person extends Animal {}
const person = new Person('ํ๊ธธ๋');
person.eat();
Dog์ Person์ Animal์ด๋ผ๋ ๊ณตํต์ ์ด ์๊ธฐ์ class Animal์ ๋ง๋ค๊ณ ๋์ด ๊ฒน์น๋ ๋ถ๋ถ์ ์ ๋ฆฌํ๋ค.
๊ทธ๋ฆฌ๊ณ extends๋ฅผ ํตํด ์์๋ฐ์ผ๋ฉด Animal์ ์๋ ๋ฉ์๋๋ฅผ Dog์ Person๋ ์ฌ์ฉํ ์ ์๋ค.
class Person extends Animal {
constructor(name, hobby) {
super(name);
this.hobby = hobby;
}
call() {
console.log(this.name, this.hobby);
}
}
const person = new Person('ํ๊ธธ๋', '๊ณต๋์ด');
person.call(); //ํ๊ธธ๋, ๊ณต๋์ด
Animal์ด ๊ฐ์ง๊ณ ์๋ name์ ์ฐ๊ณ , ์๋ก์ด hobby๋ฅผ ๋ง๋ค๊ณ ์ถ๋ค๋ฉด?
super๋ฅผ ํตํด name์ ์ฌ์ฉํ๊ณ , hobby๋ ์๋ก contructor์ ๋ฃ์ด์ค๋ค.
class Person extends Animal {
constructor(name, hobby) {
super(name);
this.hobby = hobby;
}
play() {
super.play();
console.log('๋๊ฑฐ์ผ?');
}
}
const person = new Person('ํ๊ธธ๋', '๊ณต๋์ด');
person.play();
//๋
ผ๋ค
//๋๊ฑฐ์ผ?
๋ถ๋ชจ ๋ฉ์๋๋ฅผ ํ ๋๋ก ์ผ๋ถ ๊ธฐ๋ฅ๋ง ๋ณ๊ฒฝํ๊ณ ์ถ์ ๋๋ super๋ฅผ ์ฌ์ฉํ๋ค.
์ด๋ฅผ ์ค๋ฒ๋ผ์ด๋ฉ์ด๋ผ๊ณ ํ๋ค.
์ ์ฝ๋์์ play๋ ์์๋ฐ์ Person์๋ ์กด์ฌํ๋๋ฐ super๋ฅผ ํ์ง ์๊ณ ์๋ํ๋ค๋ฉด Person.play์ธ '๋ ๊ฑฐ์ผ?'๋ง ์ถ๋ ฅ๋๋ค.
๊ทธ๋ฌ๋ super๋ฅผ ์ฌ์ฉํ๋ฉด Animal.play์ธ '๋ ผ๋ค'๋ ํจ๊ป ์ถ๋ ฅ๋จ์ ์ ์ ์๋ค.
'JavaScript' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
setTimeout๊ณผ setInterval (0) | 2023.11.02 |
---|---|
๋ฐฐ์ด (0) | 2023.02.26 |
๊ฐ์ฒด (0) | 2023.02.21 |
ํจ์ (0) | 2023.02.19 |
์ ์ด๋ฌธ (0) | 2023.02.19 |
๋๊ธ